The information in this article applies to:
Product: MIM
Version: 8.5
Platform: Windows
Discussion
This article explains the concept of invoking Microsoft COM (Component Object Model) objects via MIM XMScript. COM is Microsoft's binary interface technology that allows software components to communicate with each other and is available to applications in the Microsoft Office Family of products. The examples within this article are purely for illustration of the concept, as there are numerous COM objects that may be invoked.
In these examples, MIM invokes the COM interface to:
- Obtain operating system information
- Add a new contact to Outlook
- Add an appointment in the Outlook Calendar
- View appointments within Outlook
Prior to illustrating the use of COM objects within XMScript, the creation of a COM object instance and initialization of the COM library are explained.
Create a COM Object Instance
To create an instance of a COM object, XMScript provides a call to the createObject method, in which the programmatic identifier (ProgID) of the COM object is passed.
var refObj = createObject("ProgID", context)
The programmatic identifier is normally documented in the reference material that is delivered with the application. If documentation is not available, the ProgID may be found in the Microsoft Windows registry in the HKEY\_CLASSES\_ROOT portion of the registry. For the examples in this article, the context will be set to 4 (CLSCTX\_LOCAL\_SERVER), which indicates the executable that manages the COM object runs on the same machine, but in a separate process space.
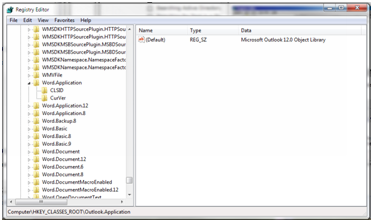
Initialize the COM library
After the reference to the COM object is stored in the variable, the script can invoke the COM object’s methods and access its attributes. However, before any COM features are accessed, the XMScript code must initialize the COM library. This will make the COM library available to all functions in the script.
CoInitialize()
Example 1: Obtain Operating System Information
Use Microsoft Word to query operating system attributes and produce a report.
function main() \{
CoInitialize();
var msWord = createObject("Word.Application",4);
msWord.Visible = 1;
var doc = msWord.documents.add();
var sel = msWord.Selection;
sel.TypeText("XMScript found Some System Parameters:");
sel.HomeKey(5, 1);
sel.Font.Bold = 1;
sel.EndKey(5, 0);
sel.Font.Bold = 0;
var tabi = doc.Tables.Add(sel.Range, 3, 2);
sel.TypeText("Operating System");
sel.MoveRight(12);
sel.TypeText(msWord.System.OperatingSystem);
sel.MoveLeft(12);
sel.MoveDown(5);
sel.TypeText("Processor");
sel.MoveRight(12);
sel.TypeText(msWord.System.ProcessorType);
sel.MoveLeft(12);
sel.MoveDown(5);
sel.TypeText("Word Version");
sel.MoveRight(12);
sel.TypeText(msWord.Version);
sel.MoveLeft(12);
sel.MoveDown(5);
return 0;
\}
Example 2: Add a Contact to Outlook
This example will add a new contact into Outlook for Joe Johnson.
function main() \{
var rc = CoInitialize();
var outlook = createObject("Outlook.Application",4);
var olContactItem = 2;
var contact = outlook.CreateItem(olContactItem);
contact.Title = "Mr";
contact.FirstName = "Joe";
contact.LastName = "Johnson";
contact.EMail1Address = -removed-;
contact.save();
return 0;
\}
Example 3: Add an Outlook Calendar Appointment
This example will add a new Outlook business presentation appointment at 3:00pm on June 17, 2010 in the boardroom.
function main() \{
var rc = CoInitialize();
var outlook = createObject("Outlook.Application",4);
var olAppointmentItem = 1;
var appointment = outlook.createItem(olAppointmentItem );
appointment.start = "06/17/2010 15:00";
appointment.subject = "Business Presentation";
appointment.duration = 120;
appointment.location = "BoardRoom";
appointment.body = "The purpose of this presentation is to provide an explanation about ...";
appointment.save();
return 0;
\}
Example 4: View Outlook Calendar Appointments
This example will iterate through the appointments in the Outlook calendar.
function main() \{
var rc = CoInitialize();
var outlook = createObject("Outlook.Application",4);
var objNameSpace = outlook.GetNamespace("MAPI");
var outlookFolderCalendar = 9;
var outlookFolder = objNameSpace.GetDefaultFolder(outlookFolderCalendar);
var oItems = outlookFolder.Items;
var oAppt = oItems.GetFirst();
var count = oItems.Count;
while(count) \{
print(oAppt.Subject);
print("Organizer: " + oAppt.Organizer);
print("Start: " + oAppt.Start);
print("End: " + oAppt.End);
print("Location: " + oAppt.Location);
print("Recurring: " + oAppt.IsRecurring);
oAppt = oItems.GetNext();
print("---------------------------------");
count --;
\}
return 0;
\}
Note, with proper error handling added, these examples will work in any Windows hosted MIM services that can invoke an XMScript, such as the XMScript Workflow Servlet Engine and the Exit Service, as well as standalone XMScripts.
---
Note: Some information in this post has been hidden to protect privacy.